An example to control the intensity of vibration using PWM for the Vibration DC Motor Module. For example this model:
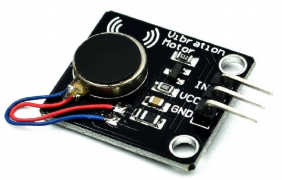
Connect the module to 5V (VCC) and ground (GND) and the IN pin to any PWM pin of the Arduino. Eg. for an Arduino Uno, this can be pin 3. For an overview of PWM pins for different Arduino models, check this overview.
The example sketch below will make the motor vibrate on full power for 2 seconds. Then for half power for 2 seconds, and then off for two seconds. And repeat this cycle (loop).
int motorPin = 3; // IN pin from motor connected to digital pin 3
// (this is a PWM pin on for instance the Arduino Uno)
void setup() {
pinMode(motorPin, OUTPUT); // sets the pin as output
}
void loop() {
analogWrite(motorPin, 255); // turn on motor on full power (range is 0 - 255)
delay(2000); // wait 2 sec., so motor will vibrate for 2 sec
analogWrite(motorPin, 125); // turn on motor on half power
delay(2000); // wait 2 sec., so motor will vibrate for 2 sec
analogWrite(motorPin, 0); // turn off motor
delay(2000); // wait 2 sec., so motor will be off for 2 sec
}
If it does not work, try changing the motorPin value from 3 to A3 or viseversa. The motorPin value being a PWM capable pin varies per Arduino board. Check that here.
It is also possible to use digitalWrite()
to control the motor. However, in that case you can not set the intensity of the vibration.