To start drawing inside a panel, first setup a DrawingPanel as described in step 2 of this assignment. Next, add a paintComponent() method and add drawing commands to it. The origin in a panel is the upper left corner:
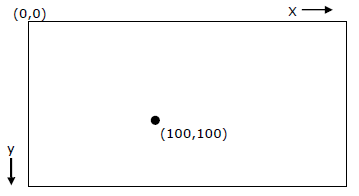
The first time you use a new class, like Color, you might get a warning bulb in the margin:
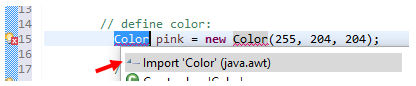
To solve, just click the bulb and double-click the first option to Import that class from the library (in this case java.awt).
The examples below do not have the line super.paintComponent(g);. You should keep that line from the generated code (as shown in the assignment). It should be the first line of code. (So add the drawing code like g.setColor… and g.draw… after it)
Draw a rectangle
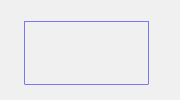
public void paintComponent(Graphics g) {
super.paintComponent(g); // this line must always be first!
// set color:
g.setColor(Color.blue);
// draw rectangle at position (60,60) w x h 200x100:
g.drawRect( 60, 60, 200, 100);
}
Draw text
public void paintComponent(Graphics g) {
super.paintComponent(g); // this line must always be first!
// set the font:
g.setFont(new Font("TimesRoman", Font.PLAIN, 20));
// set color:
g.setColor(Color.red);
// draw a String at position (10,20):
g.drawString("paint some text", 10, 20);
}
Can you: Change the size of the font? Change the position of the text?
Use Color attributes: Help-while-you-type
To find out what colors are available, just type Color followed by a dot (.):
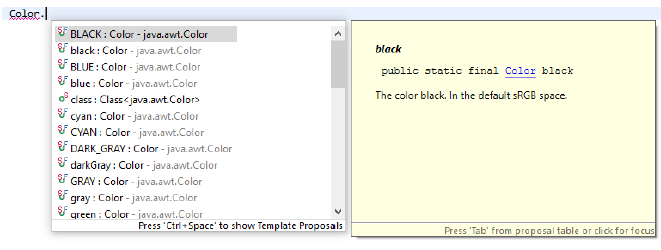
Mix your own RGB-color
Create a new color with:
new Color(255, 204, 204)
In the next example, we draw an oval using this color.
Draw an oval
public void paintComponent(Graphics g) {
super.paintComponent(g); // this line must always be first!
// define color:
Color pink = new Color(255, 204, 204);
// set color:
g.setColor( pink );
// draw oval at position (100,100) width 120, height 80:
g.drawOval(100, 100, 120, 80);
}
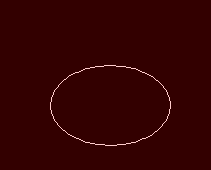
Can you: Change the oval into a circle?
Change the background
To change the background color of a JPanel, simply call the setBackground() method:
setBackground( new Color(51,0,0) );
Improve quality of drawing
You might notice that sometimes the quality of drawings is poor:
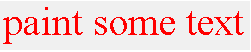
This can be improved by instructing the graphics renderer to improve the rendering quality (add this before the drawing code!):
// improve rendering by turning on Antialiasing:
Graphics2D g2 = (Graphics2D)g;
g2.setRenderingHints(new RenderingHints( RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON ));
Resulting in:

Add this code before the drawing code! More information.
Draw a filled shape
The previous shapes drawn by draw… methods each have a counterpart to draw the shape filled: those methods start with fill…
So to draw a filled rectangle we use the method fillRect():
// draw filled rectangle at position (60,60) w x h 200x100:
g.fillRect( 60, 60, 200, 100);
Draw an image
The example below draws an image (in a panel). The image is first read in the constructor of the Panel, which is called only once. This prevents the image from being read from the file each time it is (re)drawn. Copy the image to the main project folder of Eclipse.
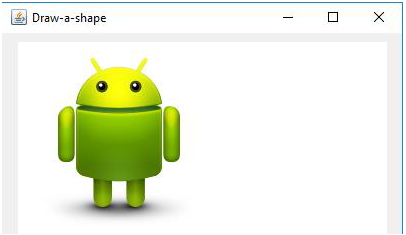
BufferedImage image; // class variable
public DrawingPanel() { // constructor
super();
File file = new File("image.png"); // read image from project-folder of Eclipse
try {
image = ImageIO.read(file);
} catch (Exception e) {
System.err.println("Unable to read file");
return;
}
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
setBackground(Color.white);
if (image != null) // if image was loaded
g.drawImage(image, 0, 0, this);
}
Draw a polygon
A polygon is a set of lines, eg. a triangle. To draw a filled triangle that fills the entire panel:
// define two lists of points, x and y, and n, which is the number of points:
int x[] = new int[3], y[] = new int[3], n =3;
// define points, we use getWidth() and getHeight() to get the width
// and height of the panel:
x[0]=0; y[0]=0; // point 1
x[1]=0; y[1]=getHeight(); // point 2
x[2]=getWidth(); y[2]=getHeight()/2; // point 3
// This polygon represents a triangle with the above vertices:
Polygon p = new Polygon(x, y, n);
// draw the polygon:
g.fillPolygon(p);
More information
Most of the methods described here are part of the Graphics class. It’s API description can be found here.